Week 3. Improving the pre proccesing
Week 3. Pre-proccesing code
for video in os.listdir(folder_path): if video.endswith(“.MP4”): video_name = video video_path = folder_path + video img_index = 0 v += 1
frames_path = folder_path + '/' + video_name + '_frames'
os.makedirs(frames_path,exist_ok=True)
cap = cv2.VideoCapture(video_path)
os.chdir(frames_path)
black_img = create_blank(w, h, rgb_color=black)
if len(dataX) != 0:
testX.append(dataX)
dataX = []
print('\nVideo #' + str(v) + '-----------' + str(video_name) + '\n')
# List all the frames
while (cap.isOpened()):
ret, frame = cap.read()
if ret == False:
break
''' FRAME RESIZED '''
frame = resize(frame, 120, 80)
''' FRAME RESIZED '''
hsv = cv2.cvtColor(frame, cv2.COLOR_BGR2HSV)
hsv = cv2.bitwise_not(hsv) # Inverted filter color
# Threshold in HSV space
lower = np.array([225, 0, 0]) # Orange
#lower = np.array([0, 0, 160]) # Green Background
#lower = np.array([0, 0, 160]) # Black background
upper = np.array([255, 255, 255])
# The black region in the mask has the value of 0
mask = cv2.inRange(hsv, lower, upper)
result = cv2.bitwise_and(frame, frame, mask = mask)
# Gray scale
gray_image = cv2.cvtColor(result, cv2.COLOR_BGR2GRAY)
# Binary Mode
ret,binary_gray = cv2.threshold(gray_image,100,255,cv2.THRESH_BINARY)
kernel = np.ones((3,3), np.uint8) # 3x3 matrix
erosion_image = cv2.erode(binary_gray, kernel, iterations=1)
kernel = np.ones((5,5), np.uint8) # 10x10 matrix
dilation_image = cv2.dilate(erosion_image, kernel, iterations=1)
# calculate moments of binary image
M = cv2.moments(dilation_image)
previous_cX = cX
if int((M["m10"]) != 0) or int((M["m10"]) != 0) or int((M["m10"]) != 0) or int((M["m10"]) != 0):
cX = int(M["m10"] / M["m00"])
cY = int(M["m01"] / M["m00"])
data_temp_x = []
data_temp_y = []
if img_index <= max_number_frames:
data_temp_x.append(np.array(cY))
data_temp_x.append(np.array(cX))
dataX.append(data_temp_x)
cv2.circle(dilation_image, (cX, cY), 3, (0, 0, 0), -1)
#cv2.putText(dilation_image, "here", (cX - 10, cY - 15),cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 0, 0), 2)
cv2.circle(frame, (cX, cY), 3, (0, 0, 0), -1)
#cv2.putText(frame, "here", (cX - 10, cY - 15),cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 0, 0), 2)
if (cX >= previous_cX):
print ('Frame#' + str(img_index) + ' centroid ----- ' + str(cX) + ' ' + str(cY))
cv2.imwrite('HVS+GREY+BIN(ERODE+DILATE) ' + str(img_index) + '.png', dilation_image)
cv2.imwrite('ORIGINAL ' + str(img_index) + '.png', frame)
cv2.circle(black_img, (cX, cY), 1, (246, 209, 81), -1)
cv2.imwrite('Real_Trails.png', black_img)
Finnally the improving of the script for extracting frames and add the centroid to the ball was working properly with the new videos recorded and use 100 fps in the camera setting instead of 50 fps
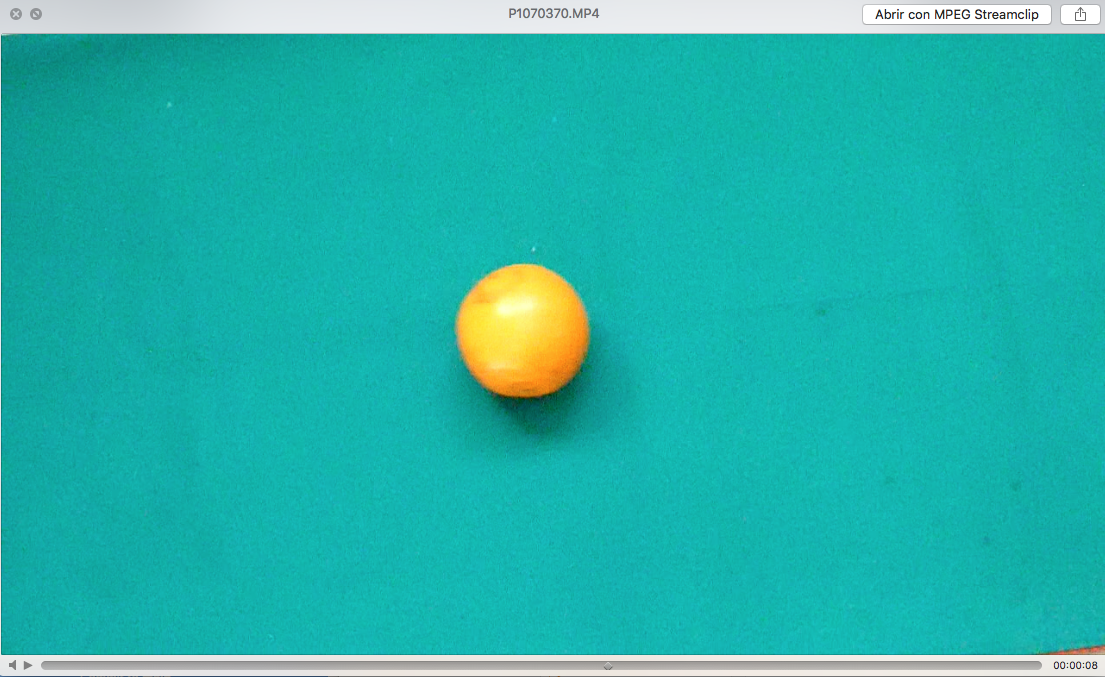
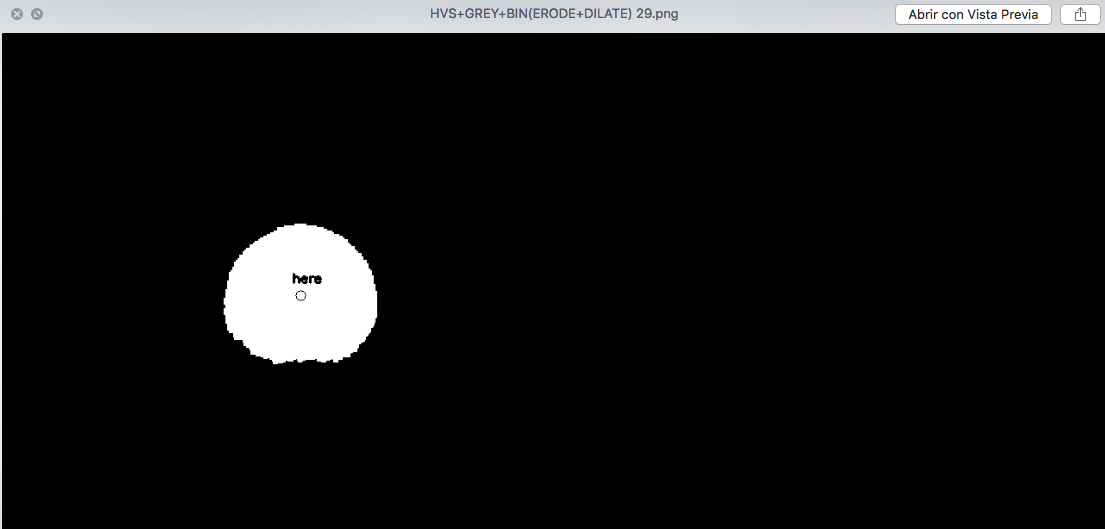
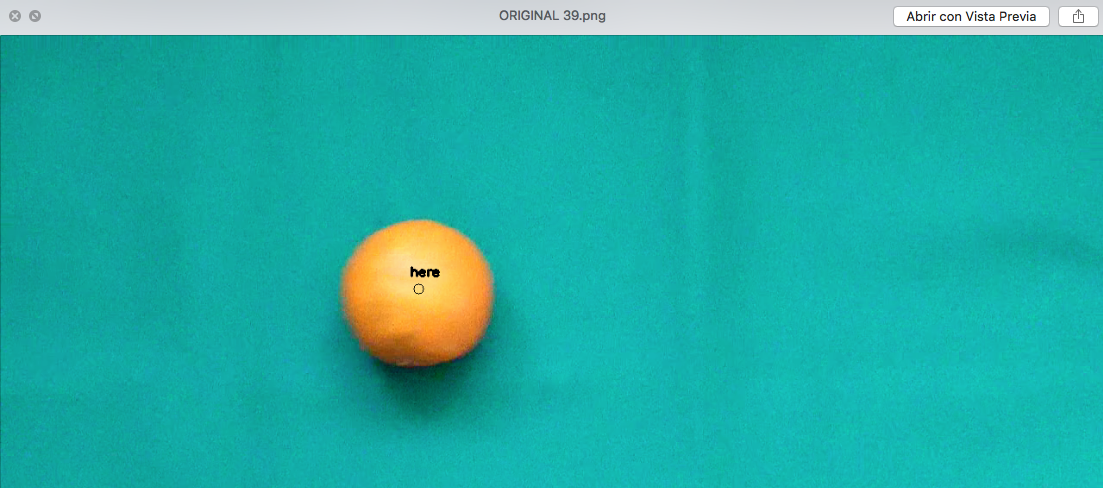